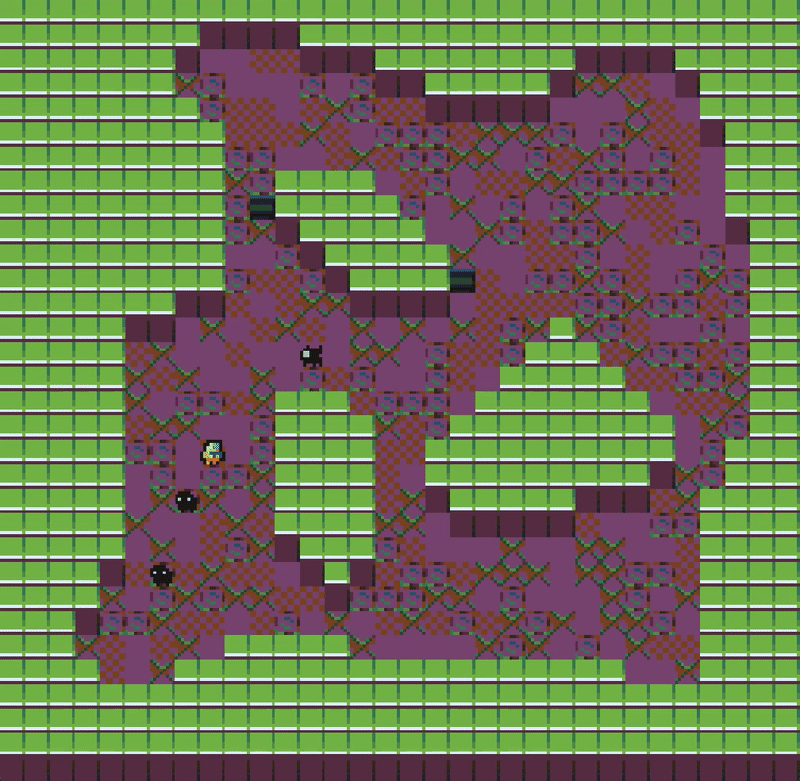
This project initially served to explore creating a game from scratch with C++ without the help of a pre-existing game engine. This is the reason that I avoided using third-party libraries where possible.
​
The engine is tile/grid-based, has a basic texture/asset loading system, reads player input and updates at a set tick rate.
Main Loop

The main loop runs in the engine's main class and calls all the other components of the engine. It calls all other components only once per tick interval, except for Input, which is called each loop, to catch the users inputs accurately.
​
Ticks make the game run at a consistent rate, independent of game performance.
​
My renderer updates last in the loop to draw the changes that have happened to the game by previous components such as the Game component that updates character positions.
Level Generator
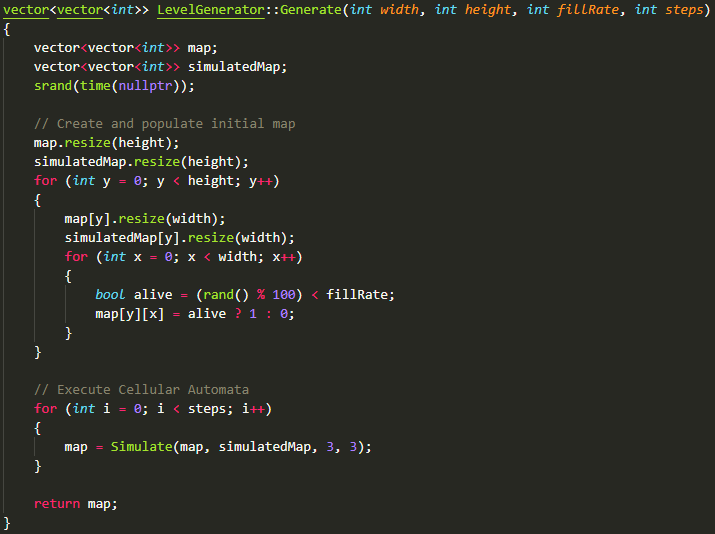


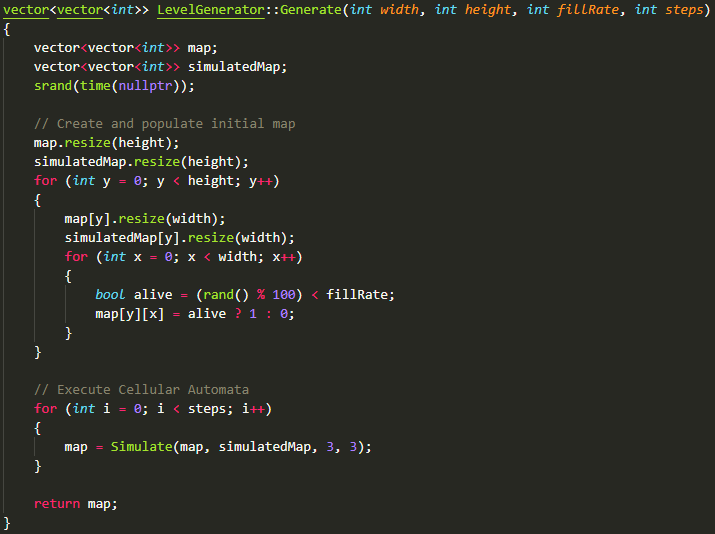
Before the game can start a level is generated with Cellular Automata
​
Generate
Creates two 2D vectors. one to represent the final result, and one for processing. The values in the map are randomized 0 and 1 values based on an initial fill rate. After this step the program calls Simulate a certain number of times to smoothen the result.
Simulate
For each tile in the grid, this counts it's neighbours and changes it's value based on the number of neighbours that have a value of 1.
This is executed stored in a separate grid to not influence the value of the current pass.
CountAdjacent
Looks at all 8 neighbours of the current tile and counts the tiles that are outside of the game grid and walls (values of 1).
Game and Input
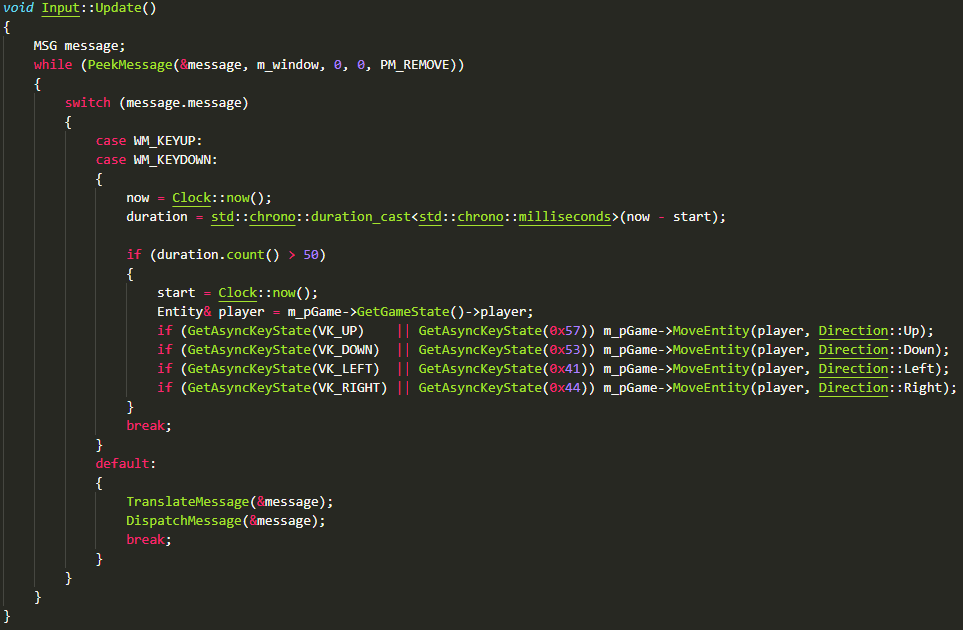
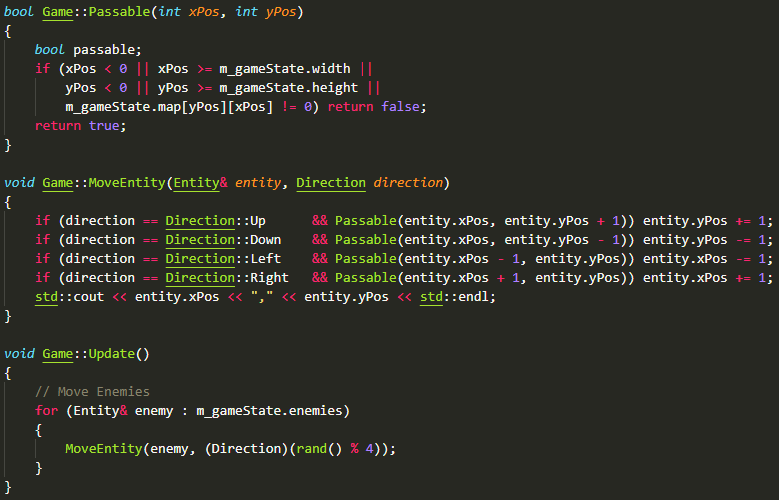
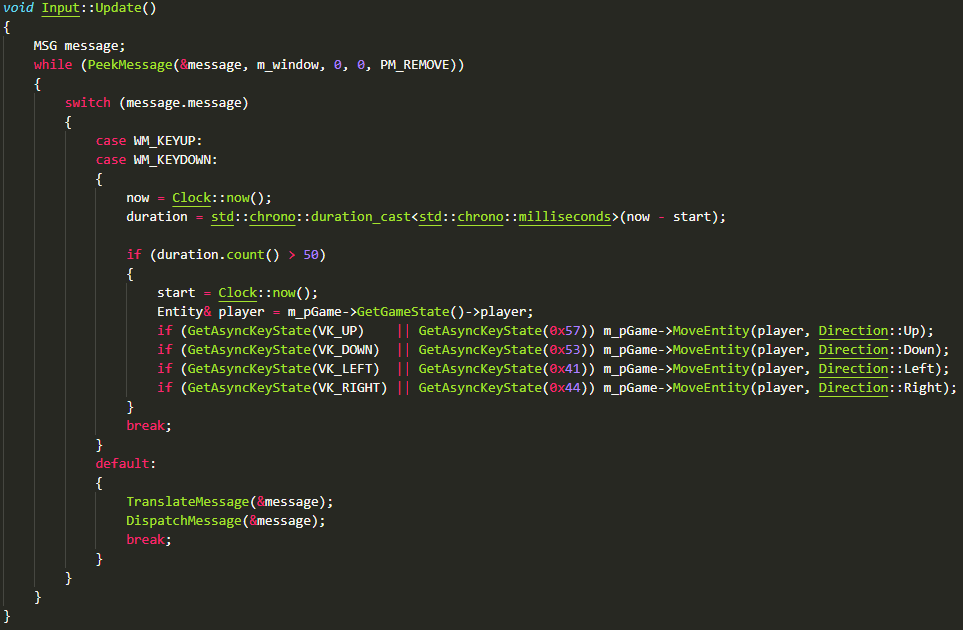
The Game component sets up the initial game state and updates monster positions each tick.
​
Rather than allowing game input to directly affect player position as it does now, i want to change input to be cached for the Game update to read. this prevents the player from moving faster than anything else in the game.
​
As the game engine grows and Entities become more advanced, they will get their own Update function that the main loop or Game component will call. Ideally every part of the engine will inherit from an interface that implements functions such as Update, similar to how an engine like Unity does it.
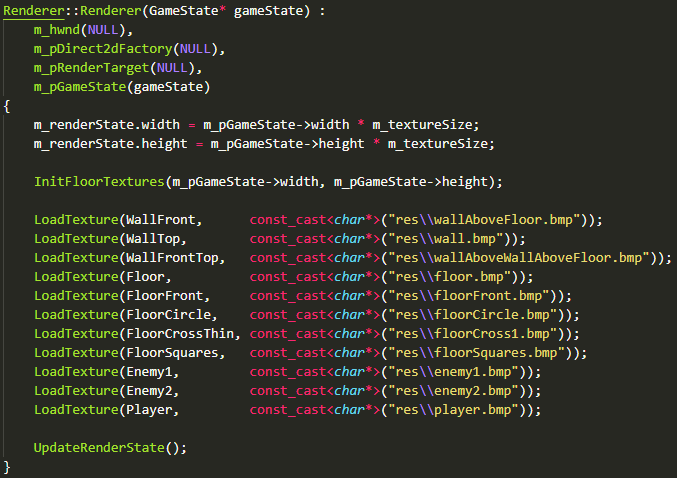
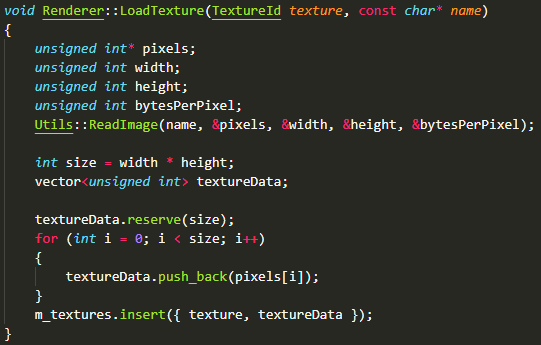
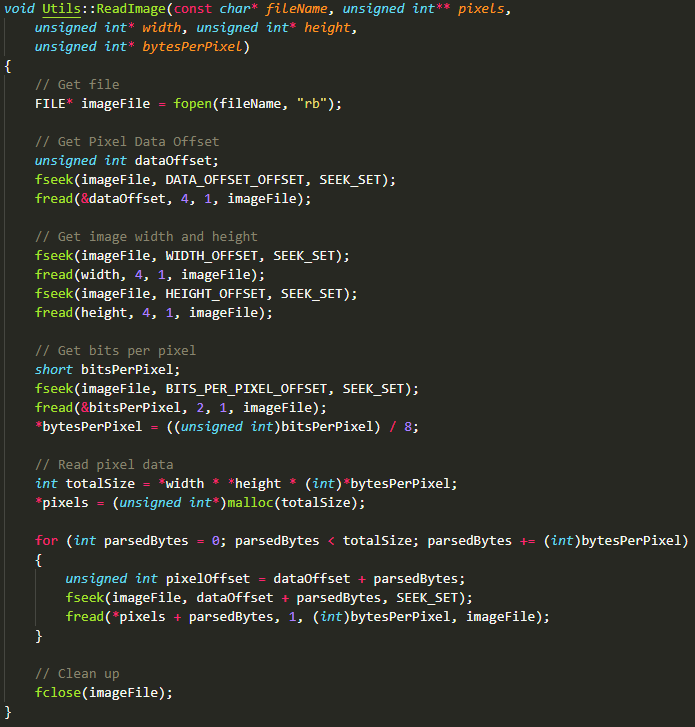
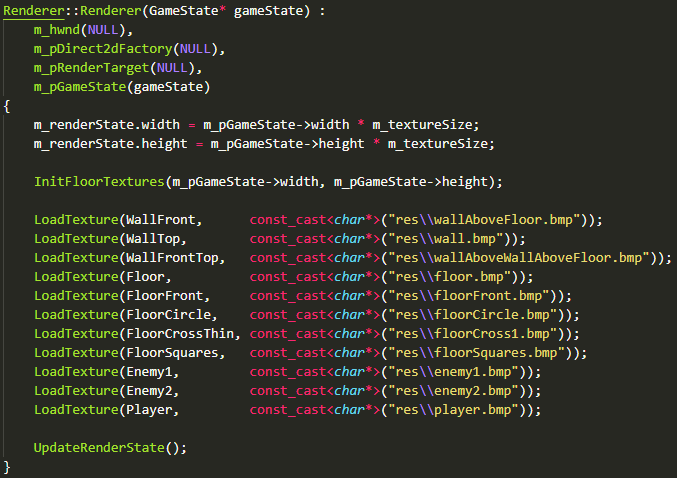
Rendering
The renderer handles drawing the current game state and changes to the window such as resizing.​
​
Asset Loading
Upon instantiation of the Renderer class i load all textures into memory as 2D color vectors. These are stored in a map where they're paired with an enum to identify them later.
​
To add variety to the floor texture i cache a 2D vector the size of the game grid and seed it with values. these can later be retrieved to determine what each floor tile should look like.
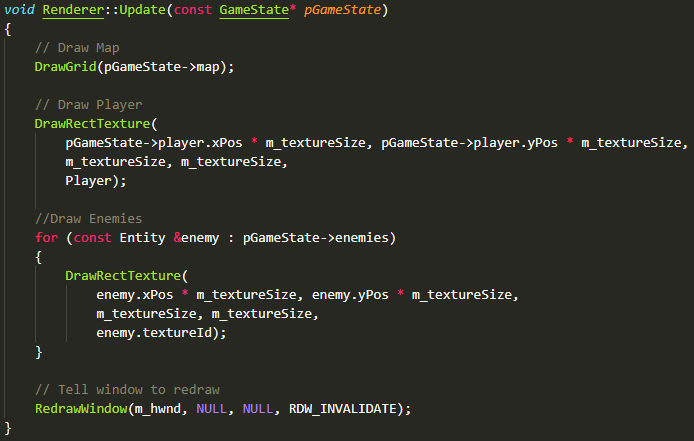
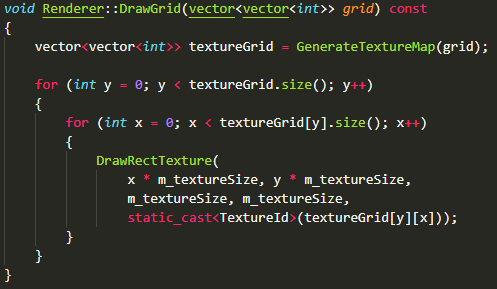
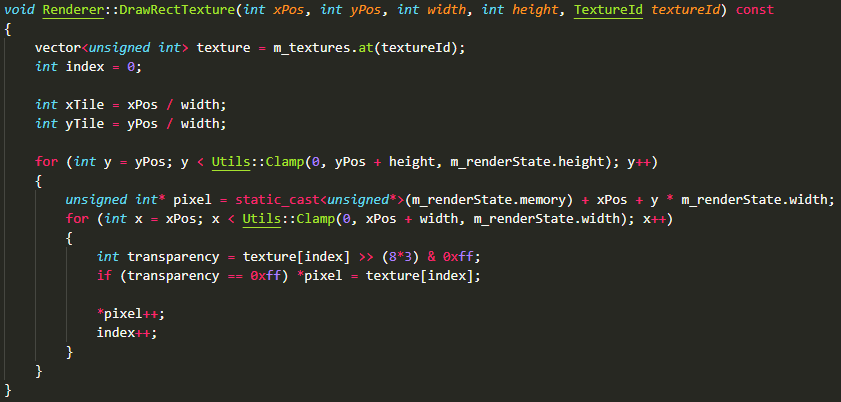
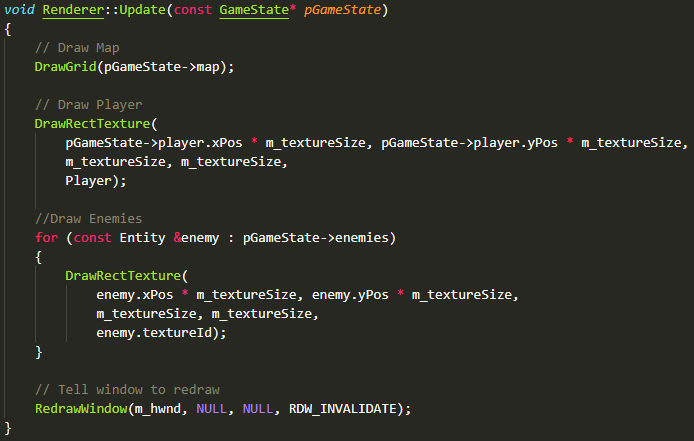
Render buffer
The renderer used a buffer that holds each pixel that is to be drawn. Other functions will replace pixels in this buffer.
​
DrawGrid steps through the entire game grid and draws the corresponding textures into the buffer using DrawRectTexture.
​
After all the changes to the buffer are done, the update loop triggers a redraw on the window, which in turn triggers the Direct2D rendering.

Direct2D
To render the game onto the application window I'm using Direct2D. I originally used GDI, but it performed very poorly on larger resolutions.
​
I convert my buffer to a bitmap and use D2D to draw the bitmap into the window.